Flutter Component Basics - GridView#
GridView is a grid layout similar to UICollectionView in iOS. It allows you to set the number of items per row, the aspect ratio of individual items, the horizontal and vertical spacing between objects, and more.
Common Properties of GridView#
- GridView
- scrollDirection: Scroll direction
- Axis.horizontal: Horizontal scrolling
- Axis.vertical: Vertical scrolling, which is the default.
- padding: The padding of GridView relative to the parent view.
- crossAxisCount: Number of items per row.
- mainAxisSpacing: Spacing between objects in the direction perpendicular to the scroll direction. For example, when scrolling horizontally, this represents the vertical spacing between objects.
- crossAxisSpacing: Spacing between objects in the direction parallel to the scroll direction. For example, when scrolling horizontally, this represents the horizontal spacing between objects.
- childAspectRatio: The aspect ratio of a single element. When scrollDirection is vertical, it represents the width-to-height ratio; when scrollDirection is horizontal, it represents the height-to-width ratio.
- scrollDirection: Scroll direction
Simple Usage#
The code is as follows:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GridView widget',
home: Scaffold(
body: GridView.count(
scrollDirection: Axis.vertical,
childAspectRatio: 2,
padding: const EdgeInsets.all(40.0),
crossAxisSpacing: 10.0,
mainAxisSpacing: 20.0,
crossAxisCount: 2,
children: <Widget>[
Container(
padding: const EdgeInsets.all(8),
child: const Text("He'd have you all unravel at the"),
color: Colors.teal[100],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text('Heed not the rabble'),
color: Colors.teal[200],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text('Sourd of screams but the'),
color: Colors.teal[300],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text('Who scream'),
color: Colors.teal[400],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text('Revolution is coming...'),
color: Colors.teal[500],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text('Revolution, they...'),
color: Colors.teal[600],
),
],
),
));
}
}
The effect is as follows:
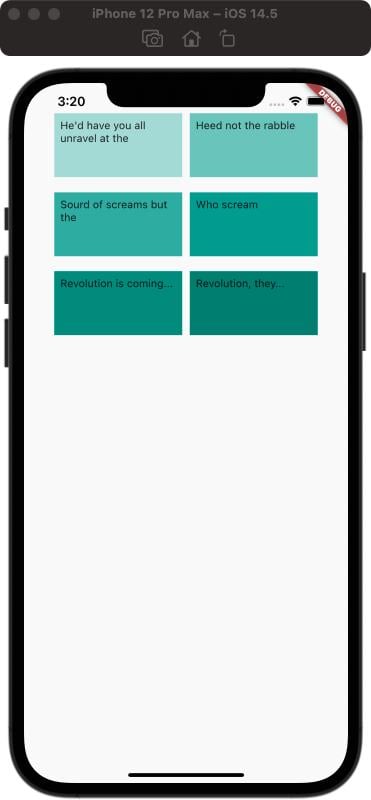
Dynamic List#
The code is as follows:
class MyGridView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GridView.builder(
padding: EdgeInsets.all(40.0),
scrollDirection: Axis.horizontal,
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 2,
mainAxisSpacing: 20.0,
crossAxisSpacing: 10.0,
childAspectRatio: 2.0),
itemCount: 6,
itemBuilder: (BuildContext context, int index) {
return Card(
color: Colors.amber,
child: Center(
child: Text('$index'),
),
);
});
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: new AppBar(
title: new Text('GridView builder'),
),
body: MyGridView(),
),
);
}
}
The effect is as follows:
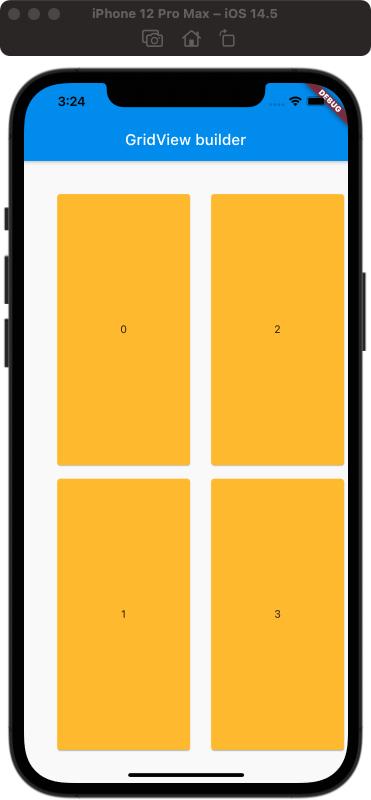
References#
GridView Dev Doc
Flutter Free Video Season 2 - Common Components